There are quite a few FormTypes available, however, we are still updating their database to be richer. It will get even bigger for the upcoming Soft-UI Theme!
Let’s introduce you to all the available ones now!
· Select
DBD.formTypes.select(list, disabled)
Argument | Type | |
---|---|---|
list | Object | Array presented as follows: { displayName: valueReturned, displayName2: valueReturned2 } |
disabled | Boolean | Boolean check for if the select should be disabled or not. |
Data required for getActualSet
function
String, valueReturned
value for option actual set.
Data returned
String, valueReturned
value for option selected.
Sample
{ optionId: 'lang', optionName: "Language", optionDescription: "Change bot's language easily", optionType: DBD.formTypes.select({"Polish": 'pl', "English": 'en', "French": 'fr'}), getActualSet: async ({guild}) => { return langsSettings[guild.id] || null; }, setNew: async ({guild,newData}) => { langsSettings[guild.id] = newData; return; } },
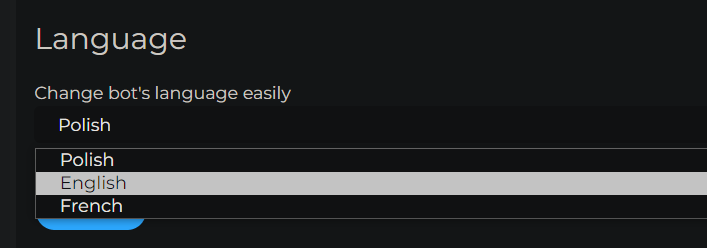
· Multiple Select
DBD.formTypes.multiSelect(list, disabled, required),
Argument | Type | |
---|---|---|
list | Object | Array presented as follows: { displayName: valueReturned, displayName2: valueReturned2 } |
disabled | Boolean | Boolean check for if the select should be disabled or not. |
required | Boolean | Boolean check for if the select should be required or not. |
Data required for getActualSet
function
Array, list of valueReturned
to be selected.
Necessary: If any, return an empty Array – no null or undefined.
Data returned
Array, list of selected valueReturned
values | or an empty Array.
Sample
{ optionId: 'multiselect', optionName: "Multi Select", optionDescription: "Select MultiValues", optionType: DBD.formTypes.multiSelect({foo: 'bar', sam: 'ple', oh: 'oh', boo: 'oob'}, false, true), getActualSet: async ({guild}) => { return multiData[guild.id] || []; // ! }, setNew: async ({guild,newData}) => { multiData[guild.id] = newData; return; } },
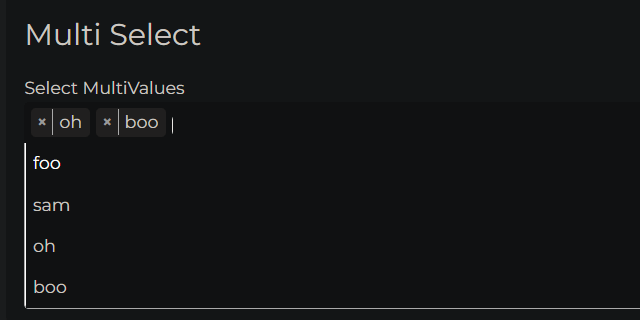
· Input
DBD.formTypes.input(placeholder, min, max, disabled, required),
Argument | Type | |
---|---|---|
placeholder | String | Input placeholder. |
min | Number | Minimum length. |
max | Number | Maximum length. |
disabled | Boolean | Boolean check for if the input should be disabled or not. |
required | Boolean | Boolean check for if the input should be required or not. |
Data required for getActualSet
function
String, value to be displayed.
Data returned
String, value set | or null.
Sample
{ optionId: 'prefix', optionName: "Prefix", optionDescription: "Set bot prefix.", optionType: DBD.formTypes.input('Prefix', 1, 4, false, false), // reqired false (if empty reset to default) getActualSet: async ({guild}) => { return prefixData[guild.id] || '!'; }, setNew: async ({guild,newData}) => { prefixData[guild.id] = newData || '!'; return; } },
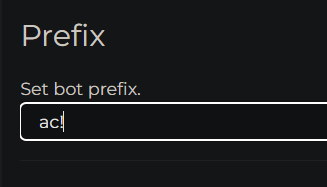
· Textarea
DBD.formTypes.textarea(placeholder, min, max, disabled, required),
Argument | Type | |
---|---|---|
placeholder | String | Textarea placeholder. |
min | Number | Minimum length. |
max | Number | Maximum length. |
disabled | Boolean | Boolean check for if the textarea should be disabled or not. |
required | Boolean | Boolean check for if the textarea should be required or not. |
Data required for getActualSet
function
String, value to be displayed.
Data returned
String, value set | or null.
Sample
{ optionId: 'longtext', optionName: "Message on member join.", optionDescription: "Set message on member join.", optionType: DBD.formTypes.textarea('Set message on member join...', null, 100, false, false), // reqired false (if empty reset to default) getActualSet: async ({guild}) => { return welcomeData[guild.id]; }, setNew: async ({guild,newData}) => { welcomeData[guild.id] = newData || null; return; } },
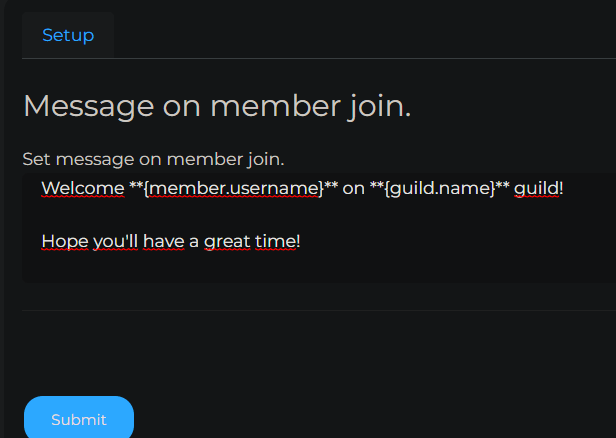
· Color Select
DBD.formTypes.colorSelect(defaultState, disabled),
Argument | Type | |
---|---|---|
defaultState | String | Default state HEX color. |
disabled | Boolean | Boolean check for if the color select should be disabled or not. |
Data required for getActualSet
function
String, actual set HEX color.
Data returned
String, new HEX color.
Sample
{ optionId: 'welcome_embed_color', optionName: "Welcome Embed Color", optionDescription: "Set embed color on member join.", optionType: DBD.formTypes.colorSelect('#fff000', false), getActualSet: async ({guild}) => { return welcomeColorData[guild.id]; }, setNew: async ({guild,newData}) => { welcomeColorData[guild.id] = newData || null; return; } },
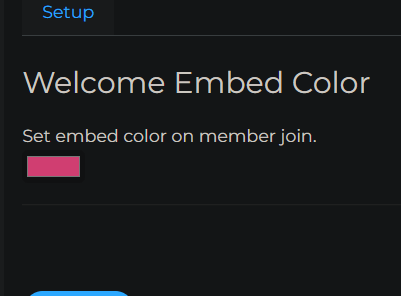
· Embed Builder
DBD.formTypes.embedBuilder(defaultSettings),
Argument | Type | |
---|---|---|
defaultSettings | Object | { username: string, avatarURL: string, defaultJson: {EMBED_BUILDER_Interface} } |
Embed Builder Interface
interface EmbedBuilder { content: string, embed: { title?: string, description?: string, color?: string | number, timestamp?: any, url?: string, author?: { name?: string, url?: string, icon_url?: string }, thumbnail?: { url?: string }, image?: { url?: string }, footer?: { text?: string, icon_url?: string }, fields?: [ EmbedBuilderField ], } } interface EmbedBuilderField { name?: string, value?: string, inline?: boolean }
Sample
{ optionId: 'welcome_embed', optionName: "Welcome Embed", optionDescription: "Build your own Welcome Embed!", optionType: DBD.formTypes.embedBuilder({ username: null, avatarURL: null, defaultJson: { content: "Did you know that if you don't know something, you don't know it? This riddle was solved by me. Don't thank me.", embed: { timestamp: new Date().toISOString(), url: "https://discord.com", description: "There was a boar, everyone liked a boar. One day the boar ate my dinner and escaped through the chimney. I haven't seen a boar since then.", author: { name: "Assistants Center", url: "https://assistantscenter.com", icon_url: "https://media.discordapp.net/attachments/911644960590270484/934513385402413076/ac_fixed.png" }, image: { url: "https://unsplash.it/380/200" }, footer: { text: "Crated with Discord-Dashboard", icon_url: "https://cdn.discordapp.com/emojis/870635912437047336.png" }, fields: [ { name: "Hello", value: "Hi, Assistants Center loves you <:ac_love:806492057996230676>" }, { name: "Do you know that", value: "You can use custom emojis there, even from server where bot isn't :Kekwlaugh:", inline: false }, ] } } }), getActualSet: async ({guild}) => { return welcomeEmbed[guild.id]; }, setNew: async ({guild,newData}) => { welcomeEmbed[guild.id] = newData || null; return; } },
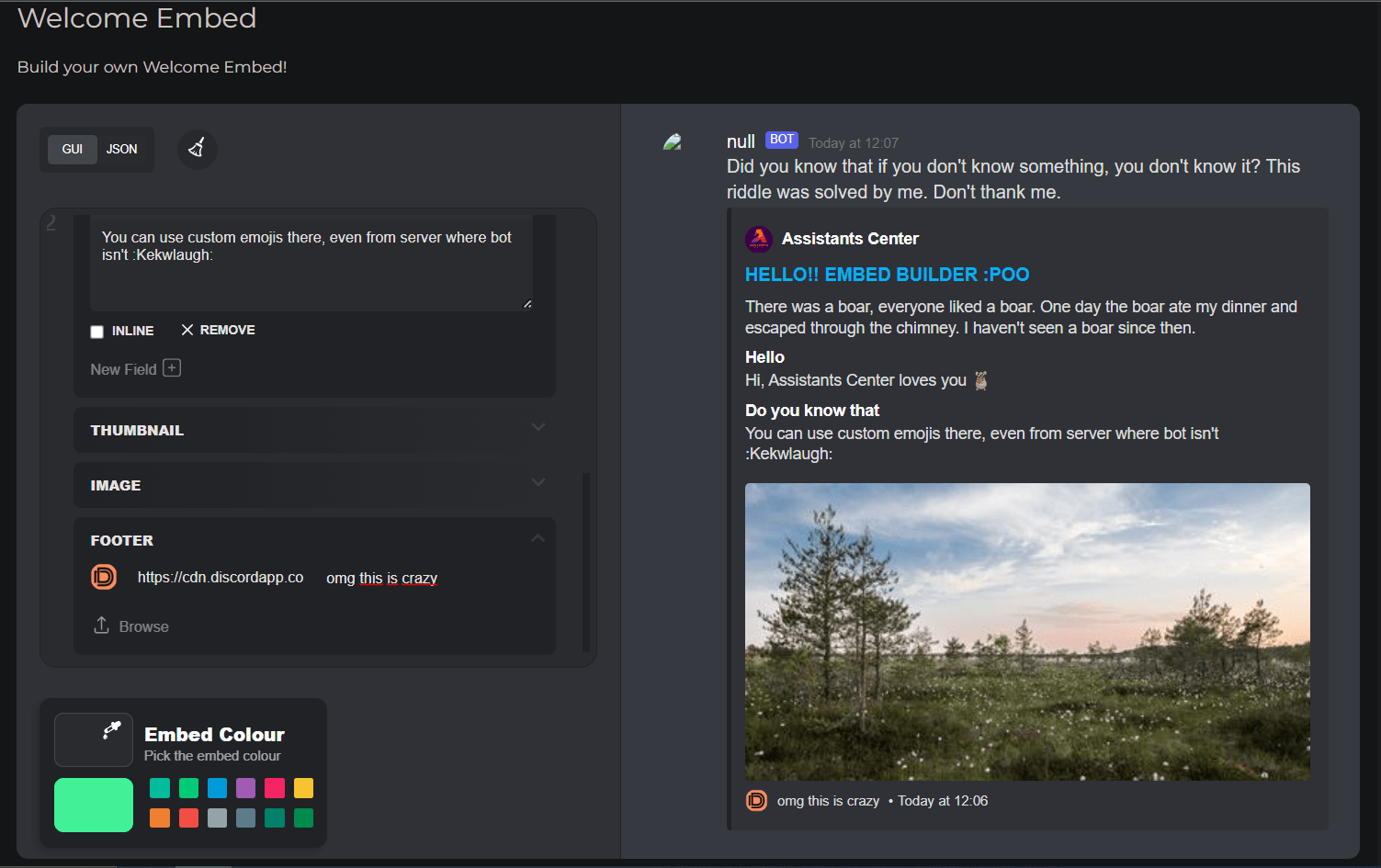
· Spacer
Usage is kinda different for this one. You don’t need to define getActualSet, etc. Just define an option as:
{ optionType: 'spacer', title: string, description: string }
Argument | Type | |
---|---|---|
title | String | Title string for spacer. You can use HTML there. |
description | String | Description string for spacer. You can use HTML there. |
Sample
{ optionType: 'spacer', title: 'Spacer!', description: 'This is just spacing and even not an option, but yeah! Cool 😎' }
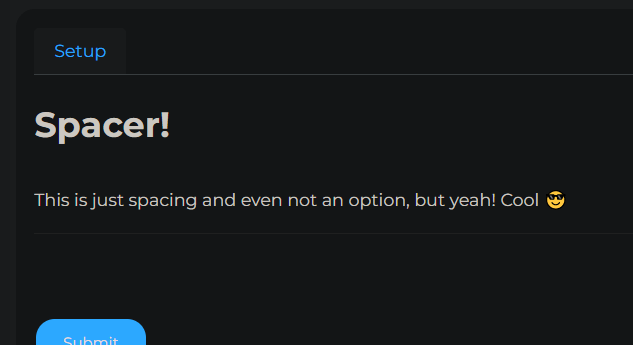
· Switch
DBD.formTypes.switch(disabled),
Argument | Type | |
---|---|---|
disabled | Boolean | Boolean check for if the switch should be disabled or not. |
Data required for getActualSet
function
Boolean, to be turned on or not.
Important:
Please note that if(saved.state)
will return false if data is not set. If you want to default state to be true, use this:
getActualSet: ({guild,user}) => { return (SAVED_STATE == null || SAVED_STATE == undefined) ? DEFAULT_STATE : SAVED_STATE; },
Data returned
Boolean, true if turned on – false if turned off.
Sample
{ optionId: 'switch_coffee', optionName: "Coffee switch", optionDescription: "Do you want coffee to be sent daily to you?", optionType: DBD.formTypes.switch(false), getActualSet: async ({guild}) => { const SAVED_STATE = switchData[guild.id]; const DEFAULT_STATE = true; return (SAVED_STATE == null || SAVED_STATE == undefined) ? DEFAULT_STATE : SAVED_STATE; }, setNew: async ({guild,newData}) => { switchData[guild.id] = newData; return; } },
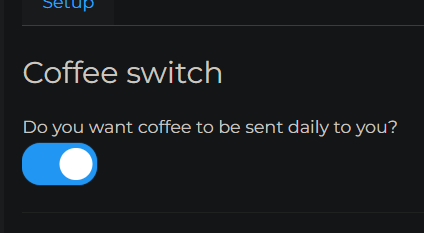
· Checkbox
DBD.formTypes.checkbox(disabled),
Argument | Type | |
---|---|---|
disabled | Boolean | Boolean check for if the checkbox should be disabled or not. |
Data required for getActualSet
function
Boolean, to be checked or not.
Important:
Please note that if(saved.state)
will return false if data is not set. If you want to default state to be true, use this:
getActualSet: ({guild,user}) => { return (SAVED_STATE == null || SAVED_STATE == undefined) ? DEFAULT_STATE : SAVED_STATE; }
Data returned
Boolean, true if ckecked – false if unchecked.
Sample
{ optionId: 'switch_coffee', optionName: "Coffee switch", optionDescription: "Do you want coffee to be sent daily to you?", optionType: DBD.formTypes.checkbox(false), getActualSet: async ({guild}) => { const SAVED_STATE = checkboxData[guild.id]; const DEFAULT_STATE = true; return (SAVED_STATE == null || SAVED_STATE == undefined) ? DEFAULT_STATE : SAVED_STATE; }, setNew: async ({guild,newData}) => { checkboxData[guild.id] = newData; return; } },
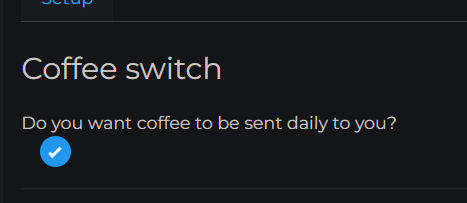
· Channels Select
DBD.formTypes.channelsSelect(disabled, channelTypes = ['GUILD_TEXT']),
Argument | Type | |
---|---|---|
disabled | Boolean | Boolean check for if the channels select should be disabled or not. |
channelTypes | Array | An Array (!) of types of channels to be included in the channels select. |
Data required for getActualSet
function
String, channel ID.
Data returned
String, channel ID | or null.
Sample
{ optionId: 'welcome_channel', optionName: "Welcome channel", optionDescription: "Select welcome message channel on this guild.", optionType: DBD.formTypes.channelsSelect(false, ['GUILD_TEXT']), getActualSet: async ({guild}) => { return welcomeChannel[guild.id]; }, setNew: async ({guild,newData}) => { welcomeChannel[guild.id] = newData; return; } },
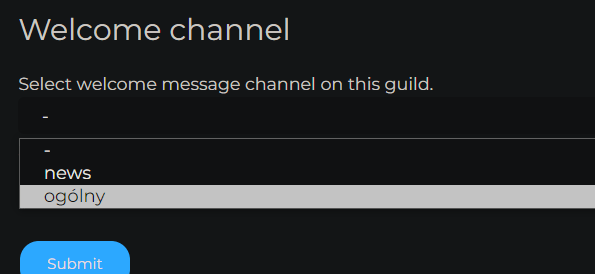
· Channels Multiple Select
DBD.formTypes.channelsMultiSelect(disabled, required, channelTypes = ['GUILD_TEXT']),
Argument | Type | |
---|---|---|
disabled | Boolean | Boolean check for if the multiple channels select should be disabled or not. |
required | Boolean | Boolean check for if the multiple channels select should be required or not. |
channelTypes | Array | An Array (!) of types of channels to be included in the multiple channels select. |
Data required for getActualSet
function
Array of Strings (Channels IDs) | or an empty Array.
Data returned
Array of Strings (Channels IDs) | or an empty Array.
Sample
{ optionId: 'multiple_channels', optionName: "Multiple Channels", optionDescription: "Select multiple channels from this guild.", optionType: DBD.formTypes.channelsMultiSelect(false, true, ['GUILD_TEXT']), getActualSet: async ({guild}) => { return multiChannels[guild.id] || []; // ! }, setNew: async ({guild,newData}) => { multiChannels[guild.id] = newData; return; } },
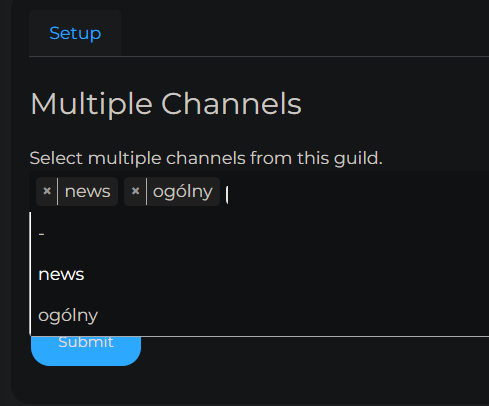
· Roles Select
DBD.formTypes.rolesSelect(disabled),
Argument | Type | |
---|---|---|
disabled | Boolean | Boolean check for if the roles select should be disabled or not. |
Data required for getActualSet
function
String, role ID.
Data returned
String, role ID | or null.
Sample
{ optionId: 'welcome_role', optionName: "Welcome role", optionDescription: "Select role given to user on guild join.", optionType: DBD.formTypes.rolesSelect(false), getActualSet: async ({guild}) => { return welcomeRole[guild.id]; }, setNew: async ({guild,newData}) => { welcomeRole[guild.id] = newData; return; } },
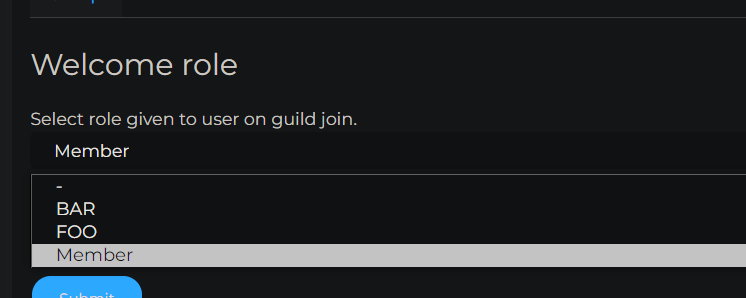
· Roles Multiple Select
DBD.formTypes.rolesMultiSelect(disabled, required),
Argument | Type | |
---|---|---|
disabled | Boolean | Boolean check for if the multiple roles select should be disabled or not. |
required | Boolean | Boolean check for if the multiple roles select should be required or not. |
Data required for getActualSet
function
Array of Strings (Roles IDs) | or an empty Array.
Data returned
Array of Strings (Roles IDs) | or an empty Array.
Sample
{ optionId: 'multiple_welcome_roles', optionName: "Multiple Welcome Roles", optionDescription: "Select multiple roles given to user on join.", optionType: DBD.formTypes.rolesMultiSelect(false, true), getActualSet: async ({guild}) => { return welcomeRoles[guild.id] || []; // ! }, setNew: async ({guild,newData}) => { welcomeRoles[guild.id] = newData; return; } },
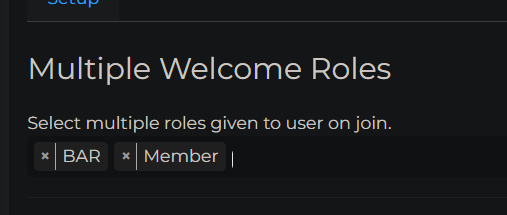
That’s it
That’s all the available FormTypes for now! Do you have any ideas or problems with something you don’t understand? Join our Discord Support Server.
We invite you to read the next parts of the tutorial.